Why You Should Write Clean Code
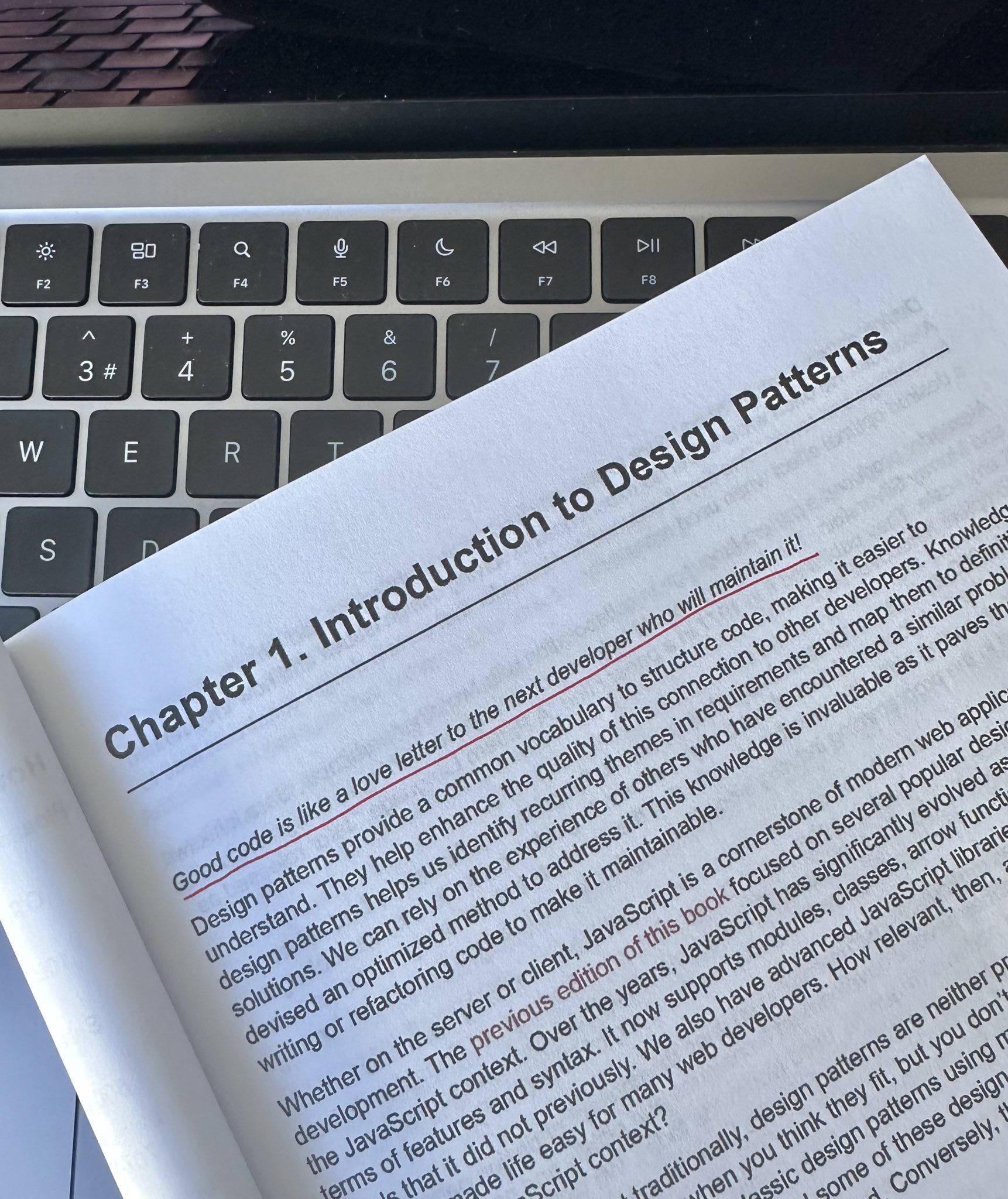
As a software developer, you probably spend a lot of time writing code. But how much time do you spend making sure that your code is clean, readable, and maintainable? If you think that clean code is not important, or that you don’t have time for it, think again. In this post, I will explain what clean code is, why it matters, and how you can write it.
What is Clean Code?
Clean code is code that is easy to understand, modify, and extend. It follows a set of principles, standards, and best practices that make it consistent, clear, and expressive. It avoids complexity, redundancy, and ambiguity. It is code that other developers can read and comprehend without much effort.
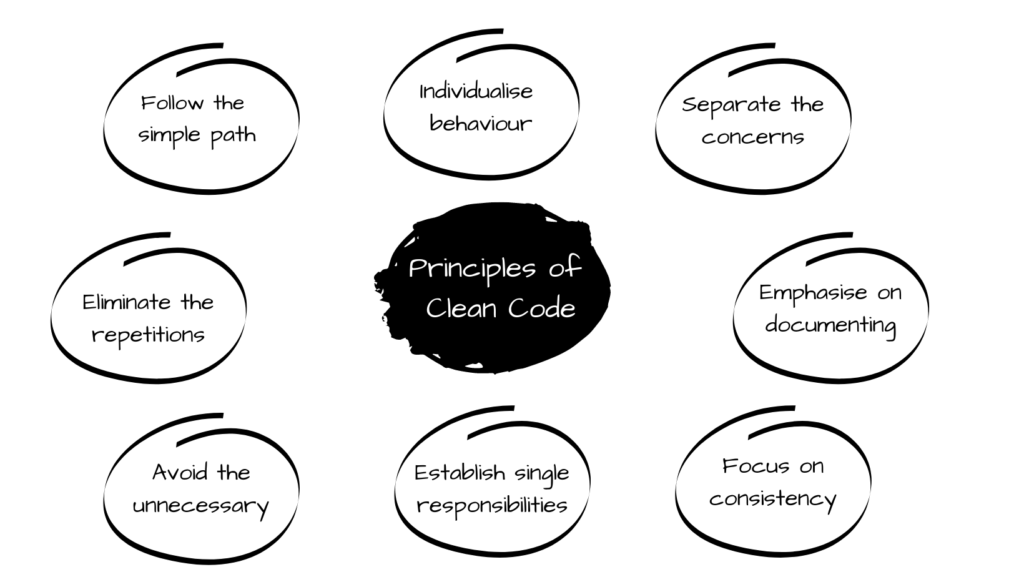
Why Does Clean Code Matter?
Writing clean code has many benefits, both for you and for your team. Here are some of them:
- It improves productivity and quality. When your code is clean, you can work faster and more efficiently. You can avoid bugs, errors, and defects that can arise from poorly written code. You can also refactor and optimize your code more easily, as well as add new features and functionalities.
- It makes collaboration and communication easier. When your code is clean, you can share it with other developers and get feedback and suggestions. You can also understand and use other developers’ code more easily. You can avoid conflicts and misunderstandings that can result from different coding styles and conventions.
- It enhances your reputation and credibility. When your code is clean, you can demonstrate your professionalism and competence. You can show that you care about your work and that you respect your colleagues and clients. You can also impress potential employers and clients with your portfolio and code samples.
How Can You Write Clean Code?
Writing clean code is not something that you can achieve overnight. It requires discipline, practice, and continuous improvement. However, there are some tips and guidelines that you can follow to make your code cleaner. Here are some of them:
- Use meaningful and consistent names. Choose names that describe the purpose and function of your variables, functions, classes, and modules. Avoid names that are too vague, too long, or too similar. Use a consistent naming convention that follows the standards of your programming language and your project.
- Write short and simple functions. Break down your code into small and focused functions that do one thing well. Avoid functions that are too long, too complex, or too nested. Use descriptive and informative names for your functions and parameters. Use comments and documentation to explain the logic and intent of your functions.
- Follow the DRY (Don’t Repeat Yourself) principle. Avoid duplicating code that performs the same or similar tasks. Extract common and reusable code into separate functions, classes, or modules. Use abstraction and inheritance to avoid code repetition and increase code reusability.
- Write clear and consistent code. Use a consistent indentation, spacing, and formatting style that follows the standards of your programming language and your project. Use meaningful and consistent symbols and operators. Use parentheses and brackets to group expressions and statements. Use comments and documentation to explain the logic and intent of your code.
- Write testable and maintainable code. Write code that can be easily tested and verified. Use unit tests, integration tests, and end-to-end tests to check the functionality and performance of your code. Use tools and frameworks that help you automate and simplify testing. Write code that can be easily modified and extended. Use modular and decoupled code that follows the single responsibility principle and the open-closed principle.
Writing clean code is not only a good practice, but also a necessity for any software developer. It can help you improve your productivity and quality, as well as your collaboration and communication with other developers. It can also help you enhance your reputation and credibility, as well as your satisfaction and enjoyment of your work.
By following the tips and guidelines in this post, you can start writing cleaner code today. Happy coding!