Exploring Next.js 13: A Game-Changer in Web Development
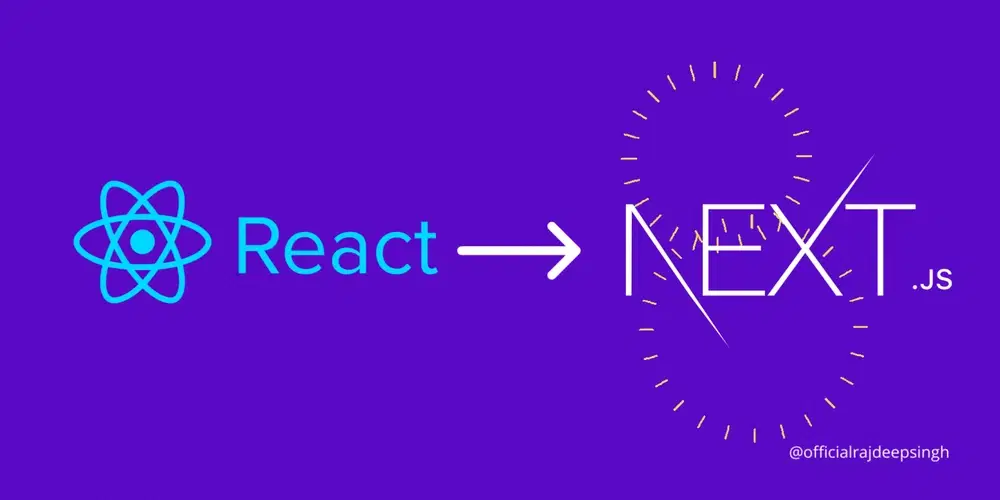
Introduction:
Next.js, the popular React framework for building server-rendered React applications, has been a game-changer in the world of web development. With its latest release, Next.js 13, there are exciting new features and improvements that web developers need to be aware of. In this blog post, we’ll dive into Next.js 13 and explore its key features, benefits, and how it can enhance your web development workflow. So, whether you’re a seasoned Next.js developer or just getting started, let’s explore the latest and greatest in Next.js 13!
What’s New in Next.js 13:
Next.js 13 brings a host of new features and improvements that make it even more powerful and efficient for building modern web applications. Let’s take a closer look at some of the key highlights of Next.js 13.
Code Splitting and Dynamic Imports:
One of the major improvements in Next.js 13 is the enhanced code splitting and dynamic imports feature. This allows you to load only the code that is needed for a particular page or component, reducing the initial load time of your application and improving performance. With Next.js 13, you can easily create dynamic imports using the import() function, making your application more optimized and efficient.
Next.js Image Optimization:
Images play a crucial role in web development, and Next.js 13 introduces powerful image optimization features. With the new Image component, you can easily optimize and serve images in different formats, such as WebP, AVIF, and JPEG, based on the browser’s support. This can significantly improve the loading speed of your web pages and provide a better user experience.
Improved Development Experience:
Next.js 13 brings several enhancements to the development experience. The new next dev command provides a local development environment with live preview, allowing you to see changes in your code in real-time without having to refresh the page. This can greatly streamline your development workflow and increase productivity.
Next.js Live Preview:
Next.js 13 introduces the Next.js Live Preview feature, which allows you to preview changes to your application in a separate window as you code. This makes it easier to see the impact of your changes in real-time and iterate quickly, without needing to constantly switch back and forth between different windows or tabs.
Enhanced Performance:
Performance is crucial for web applications, and Next.js 13 focuses on improving performance in multiple areas. With features like automatic static optimization, server components, and optimized image serving, Next.js 13 helps you build faster, more efficient, and highly performant web applications.
SEO Best Practices:
Next.js 13 also includes built-in SEO optimizations to help improve the search engine visibility of your web pages. With features like automatic generation of robots.txt and sitemap.xml files, customizable document <head> tags, and support for Open Graph and Twitter cards, Next.js 13 makes it easy to implement SEO best practices and boost your website’s search engine rankings.
Server-side Rendering (SSR) with Next.js:
One of the key features of Next.js is its ability to perform server-side rendering (SSR) out of the box. SSR allows you to generate dynamic HTML on the server and send it to the client, which provides a faster initial page load and improved SEO. With Next.js 13, SSR has been further optimized, allowing you to create blazing-fast web applications that deliver a seamless user experience.
Here’s an example of how you can implement SSR in Next.js 13:
// pages/index.js
import React from “react”;
const HomePage = ({ data }) => {
return (
<div>
<h1>Hello, Next.js 13!</h1>
<p>{data}</p>
</div>
);
};
export async function getServerSideProps() {
const res = await fetch(“https://api.example.com/data”);
const data = await res.json();
return {
props: {
data,
},
};
}
export default HomePage;
In the above code snippet, we’re using the getServerSideProps function provided by Next.js to fetch data on the server and pass it as props to the HomePage component. This way, the data is pre-rendered on the server and sent to the client as part of the initial HTML, resulting in faster page loads and improved SEO.
Static Site Generation (SSG) with Next.js:
Another powerful feature of Next.js is static site generation (SSG), which allows you to generate static HTML files at build time that can be served by a CDN for lightning-fast page loads. With Next.js 13, SSG has been further optimized, providing improved performance and scalability for your web applications.
Here’s an example of how you can implement SSG in Next.js 13:
// pages/blog/[slug].js
import React from “react”;
const BlogPost = ({ post }) => {
return (
<div>
<h1>{post.title}</h1>
<p>{post.content}</p>
</div>
);
};
export async function getStaticPaths() {
// Fetch blog post slugs from API or database
const slugs = await fetch(“https://api.example.com/blog/posts”);
const paths = slugs.map((slug) => ({
params: { slug },
}));
return {
paths,
fallback: false,
};
}
export async function getStaticProps({ params }) {
// Fetch blog post data from API or database based on the slug
const res = await fetch(“https://api.example.com/blog/posts/${params.slug}”);
const post = await res.json();
return {
props: {
post,
},
};
}
export default BlogPost;
In the above code snippets, we have a ServerComponent and a ClientComponent. The ServerComponent contains server-side logic and is rendered on the server, while the ClientComponent contains client-side logic and is rendered on the client. This way, you can optimize the performance of your web applications by offloading server-side logic to the server components and reducing the amount of JavaScript that needs to be sent to the client.
Server Components
Next.js 13 introduces an exciting new feature called Server Components, which allows developers to offload server-side logic to the server, reducing the amount of JavaScript that needs to be sent to the client and optimizing performance and scalability.
What are Server Components in Next.js 13?
Server Components are a new addition to the Next.js ecosystem that provides a way to encapsulate server-side logic in a component that is rendered on the server. This allows you to offload server-side logic, such as data fetching or API calls, to the server, reducing the amount of JavaScript that needs to be sent to the client and optimizing the performance and scalability of your web applications.
Server Components are similar to regular React components, but with some key differences. Server Components are rendered on the server and are not sent to the client as JavaScript code. Instead, they are rendered as plain HTML, which makes them highly efficient and scalable. Server Components can be used in conjunction with other Next.js features like Static Site Generation (SSG) or Server-Side Rendering (SSR) to further optimize the performance of your web applications.
Using Server Components with a Fetch Example:
// components/ServerComponent.js
import React, { useState, useEffect } from “react”;
const ServerComponent = () => {
const [posts, setPosts] = useState([]);
useEffect(() => {
const fetchData = async () => {
try {
const response = await fetch(
“https://jsonplaceholder.typicode.com/posts”
);
const data = await response.json();
setPosts(data);
} catch (error) {
console.error(“Error fetching data:”, error);
}
};
fetchData();
}, []);
return (
<div>
<h2>Server Component</h2>
<ul>
{posts.map((post) => (
<li key={post.id}>{post.title}</li>
))}
</ul>
</div>
);
};
export default ServerComponent;
In the above code, we’re using a Server Component called ServerComponent that fetches data from the “https://jsonplaceholder.typicode.com/posts” API using the fetch function. The fetched data is then rendered as a list of post titles in the component.
Next, let’s use the ServerComponent in our main component and see how it can be rendered on the client.
// pages/index.js
import React from “react”;
import ServerComponent from “../components/ServerComponent”;
const Home = () => {
return (
<div>
<h1>Welcome to Next.js 13 Server Components!</h1>
<ServerComponent />
</div>
);
};
export default Home;
Conclusion:
Next.js 13 is a game-changer in the world of web development, with its advanced features and optimizations for server-side rendering (SSR), static site generation (SSG), server components, and client components. With Next.js 13, you can create blazing-fast web applications that deliver a seamless user experience while optimizing performance and scalability. So why wait? Dive into the exciting world of Next.js 13 and unleash the power of modern web development!