Introducing the Exciting New Features in Dart 3.0
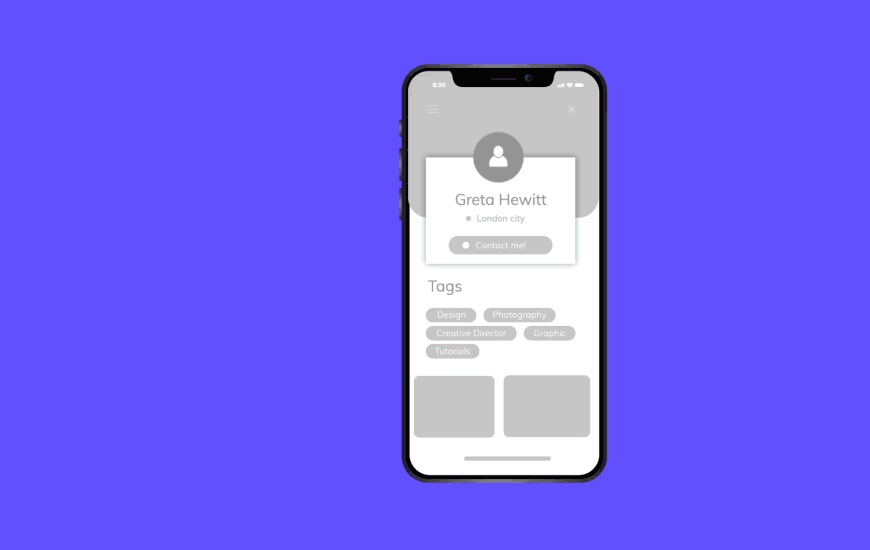
Patterns
According to documentation:
In general, a pattern may match a value, destructure a value, or both, depending on the context and shape of the pattern.
What can we do with Patterns? Let’s deep dive into the examples.
1. We can get elements of the list:
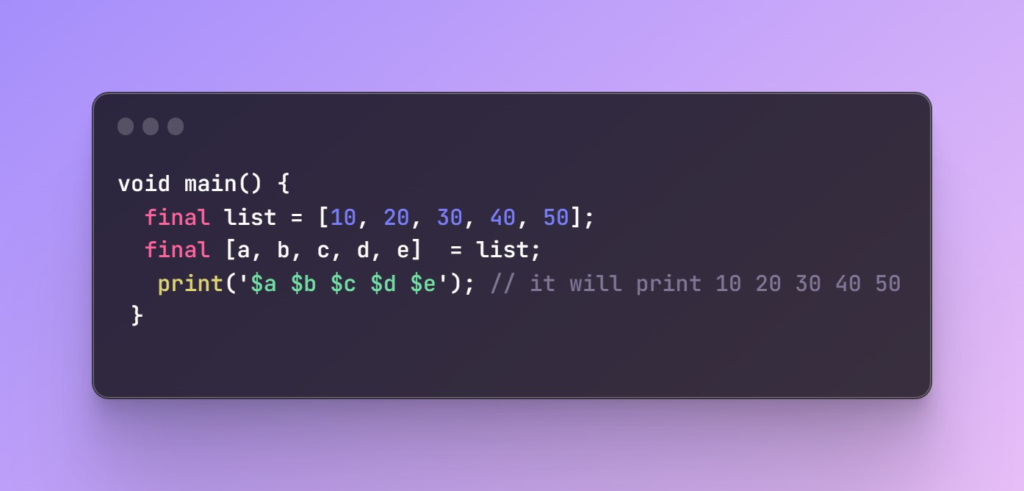
2. We can write more complex patterns and destructering cases in switch:
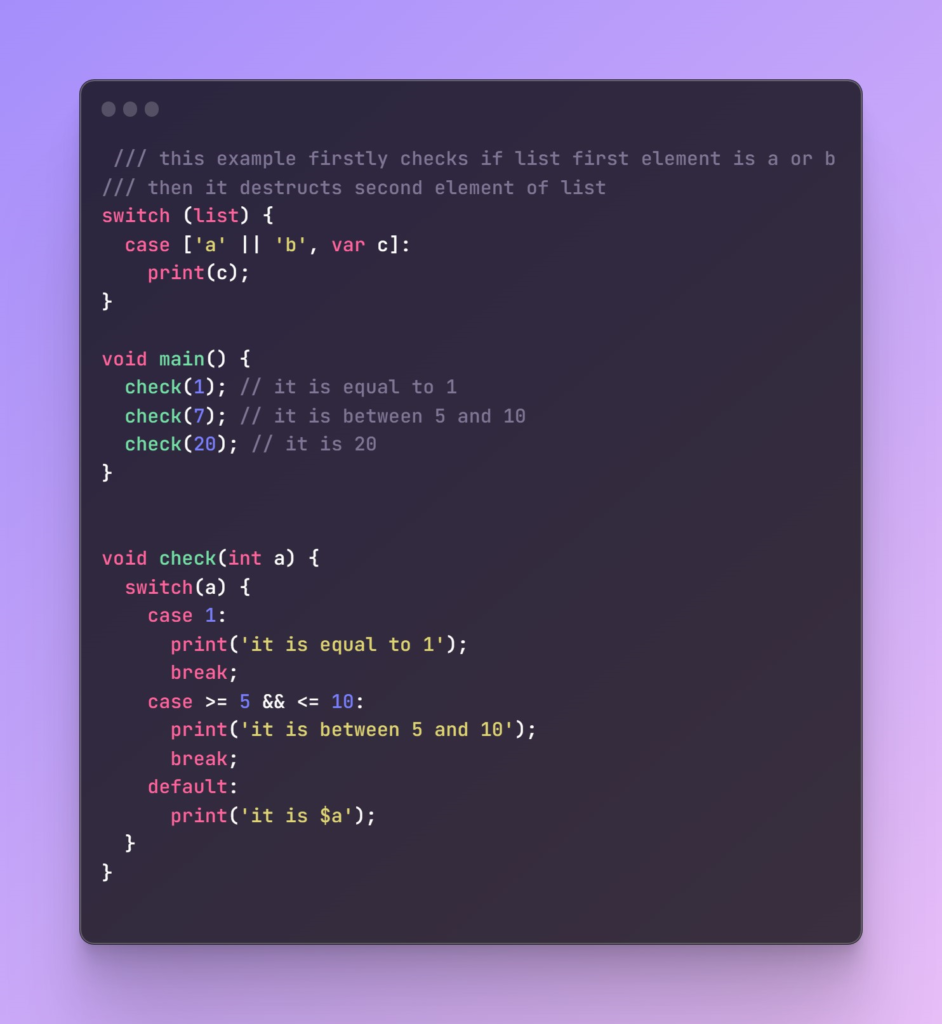
3. We can validate json in a more readable way:
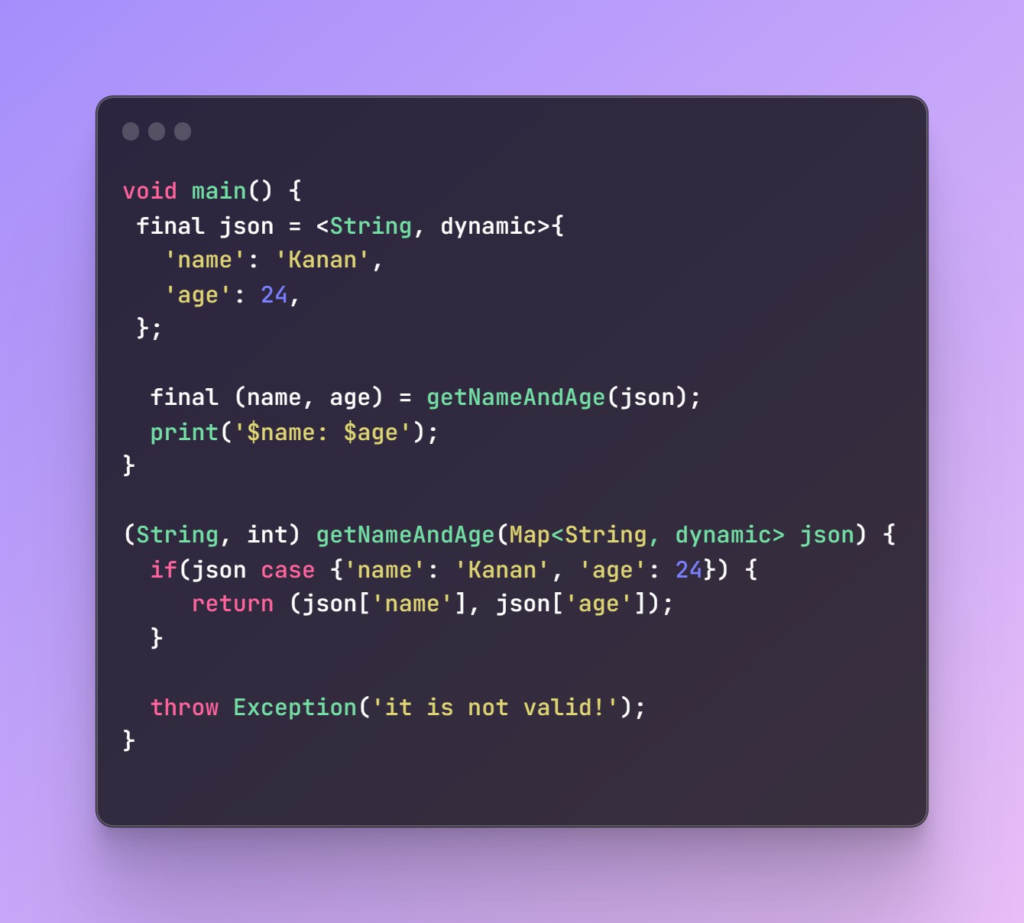
4. We can use patterns in for and for-in loops:
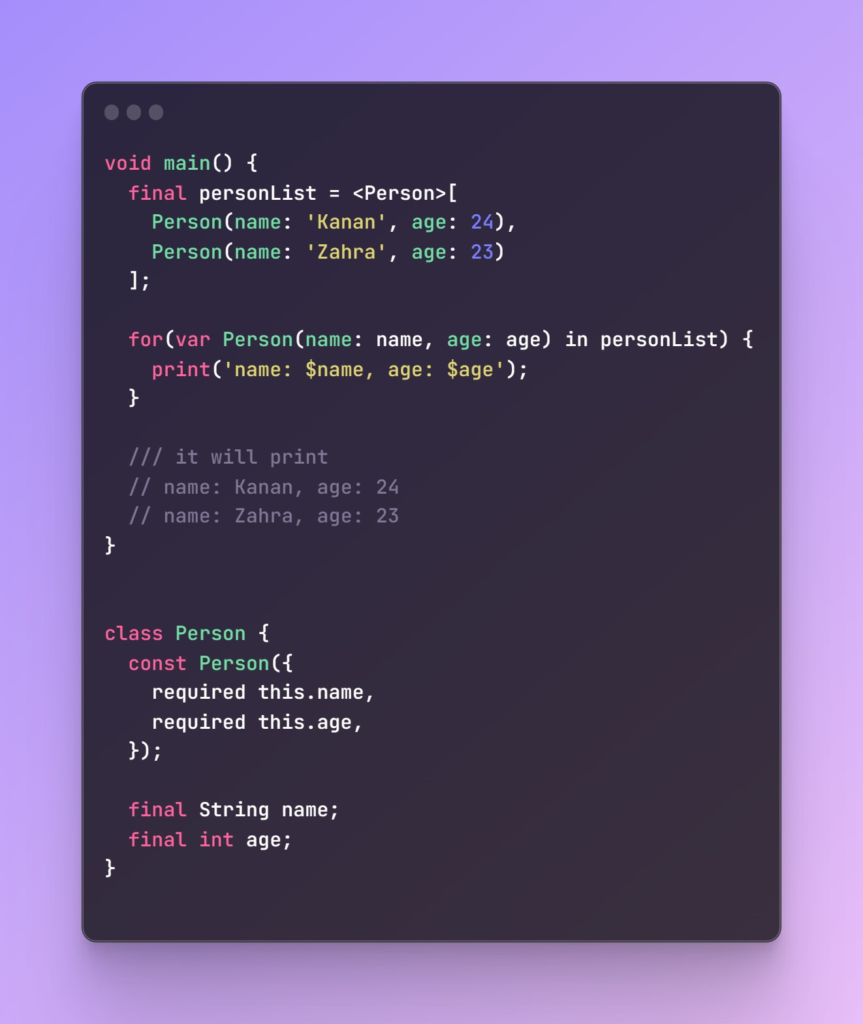
For more information, check docs.
Records (We can say tuples)
In some cases, we can encounter the case that we want to return multiple results from the method, or we want to parse JSON for only two or more fields. In these cases, we can use records. Let’s look at the following examples.
We can simply define tuples with optional or named parameters and access with its name or $index+1 way.
void main() {
var person = (name: ‘Mahedi’, surname: ‘Hasan’, age: 26);
var person2 = (‘Karan’, ‘Jhala’, 28);
print(person.age);
print(person.surname);
print(person2.$2);
}
We can use records and function parameters and return values:

We can use them as named parameters in functions too:
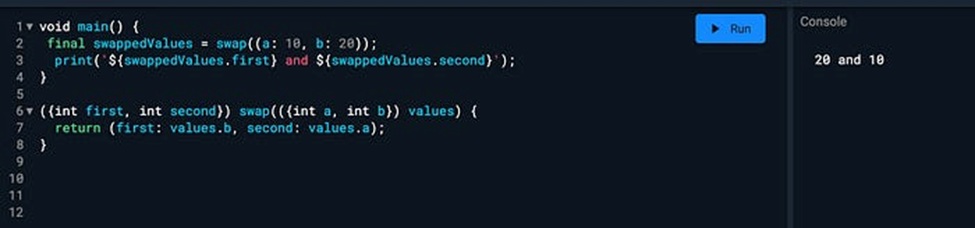
You can use it to parse some members of JSON:
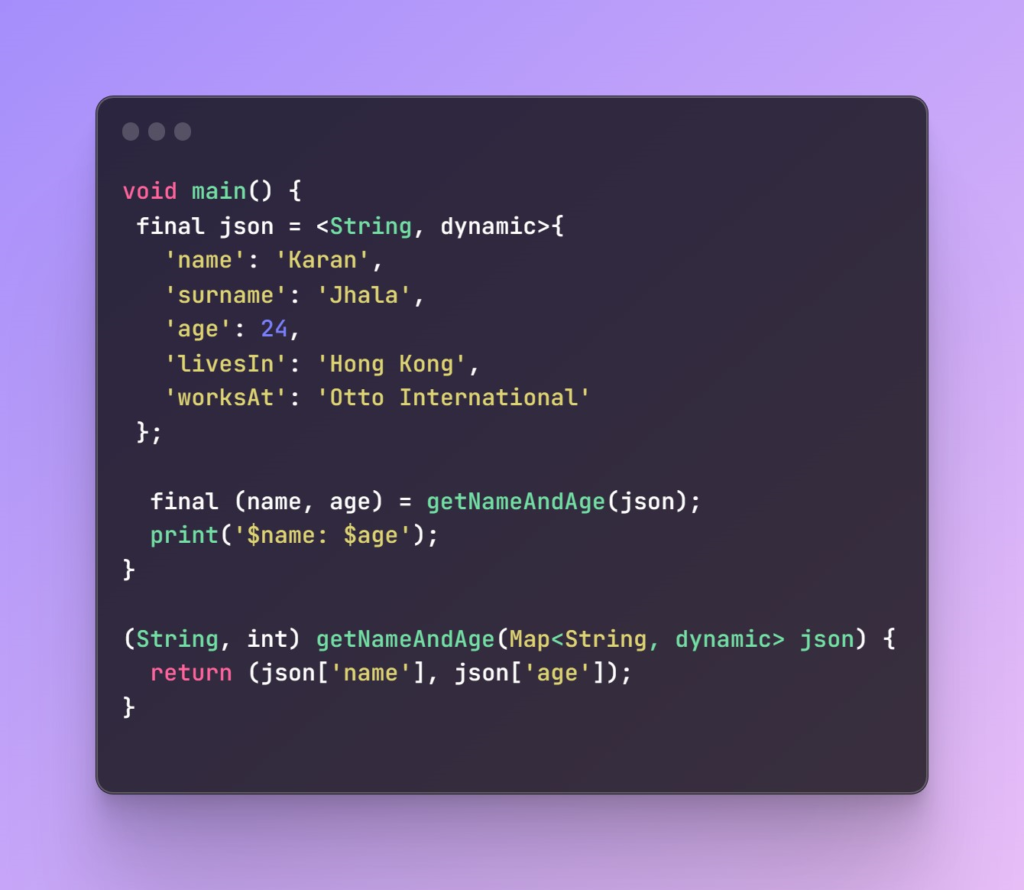
For more information, check the docs.
Class Modifiers
Final Modifier
Using this keyword in the class declaration, it will guarantee:
- Other classes can’t extend or implement your class
- Other classes can’t use this class as a mixin
Look at the following example:
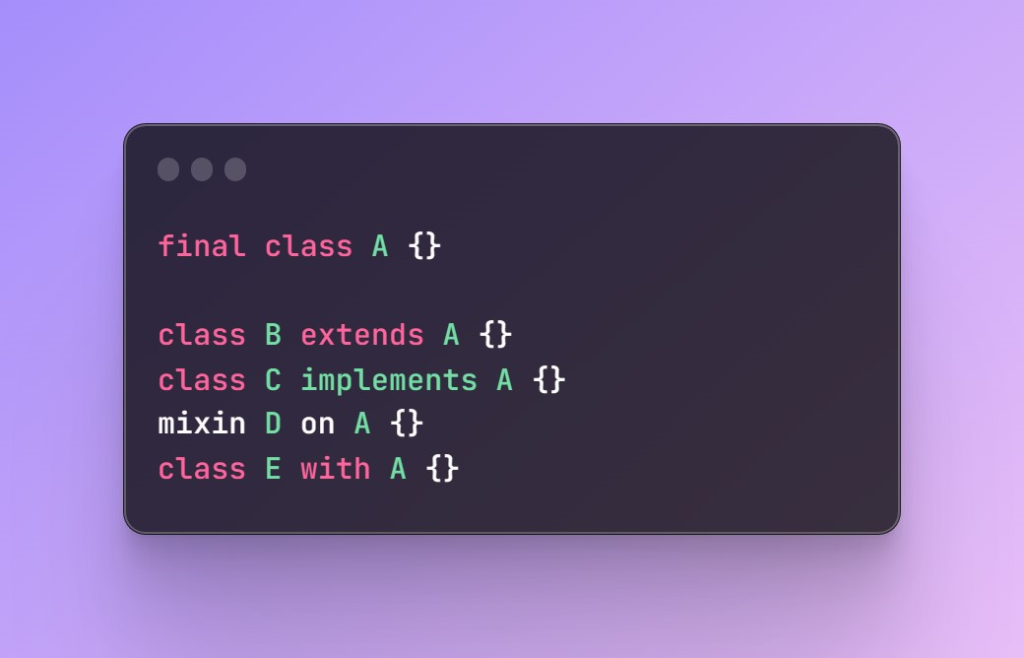
All of the above examples will give the syntax error. It guarantees that:
- You can safely add new changes to your library, and API more safely
- You can use the final class of any other library safely because it guarantees that it can not be extended or implemented in any other library.
But keep in mind that, you can extend and implement your final class within the same library. For that, you can set the subtype class as base, final or sealed.
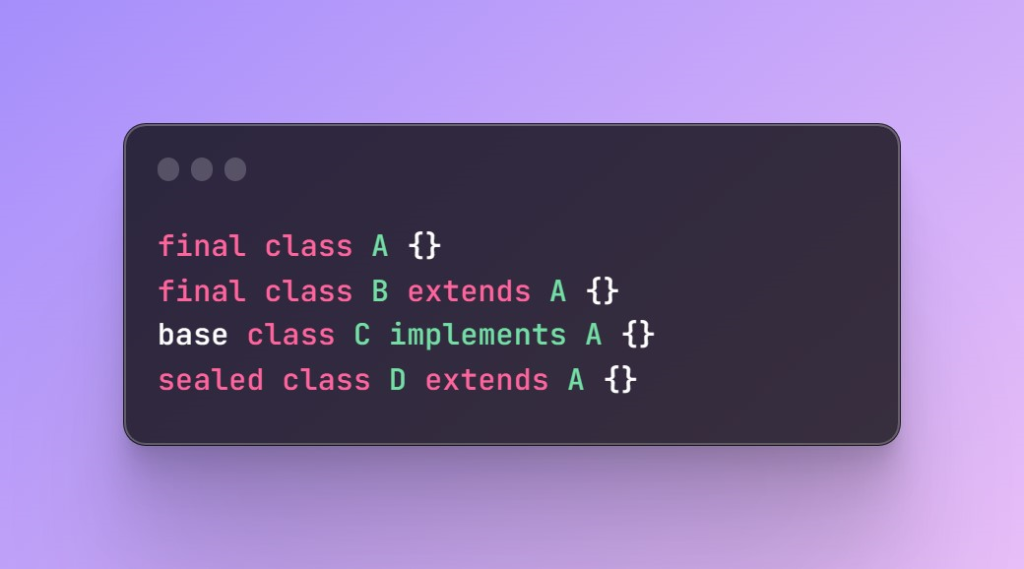
Interface Modifier
If you develop the new library, package and other things, within the same library, we can use the interface class as extended, and implemented. But if we use this library in another library, the interface class will allow us only to implement it.
So, using this modifier:
- Your library will safely call the same implementation of instance methods within the same library.
- Other libraries can’t modify the base class definition for its instance methods. It will reduce the risk of fragile base class problem.
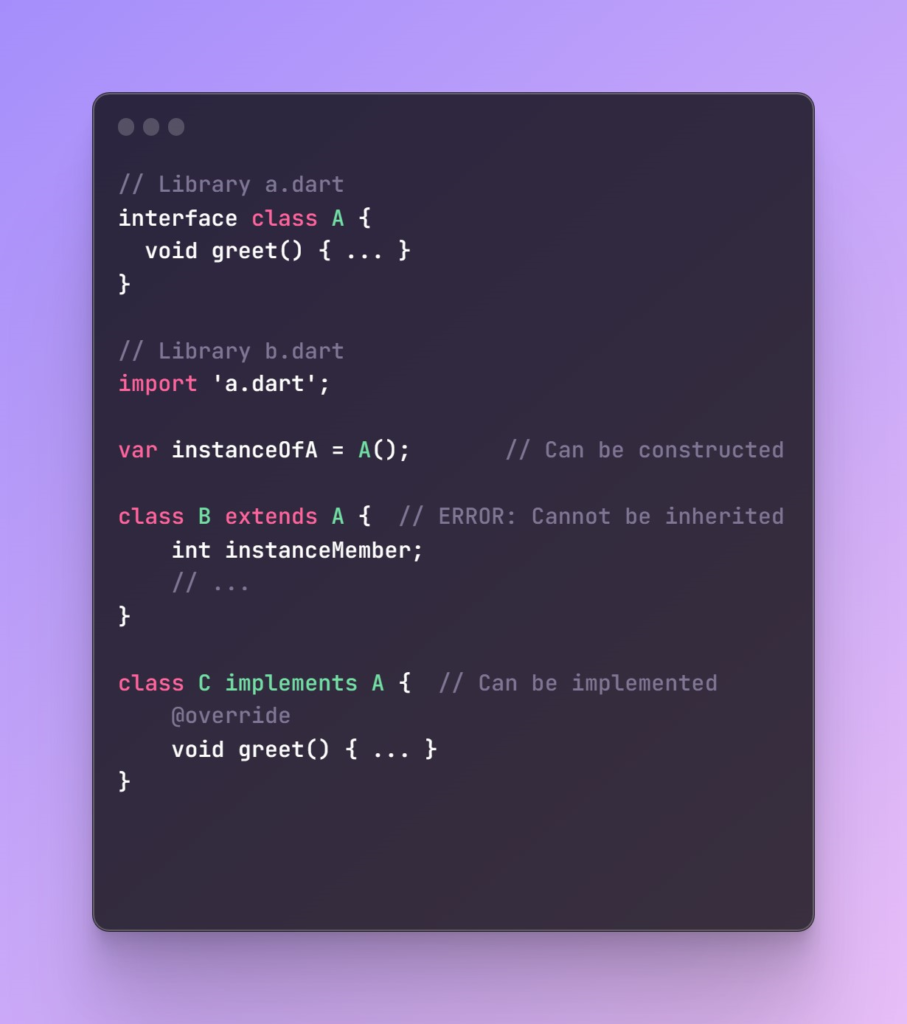
Base Modifier
Base modifier guarantees:
- The base class constructor is called whenever an instance of a subtype of the class is created.
- All implemented private members exist in subtypes.
- A newly implemented member in a base class does not break subtypes, since all subtypes inherit the new member.
- This is true unless the subtype already declares a member with the same name and an incompatible signature.
Let’s explore the following example:
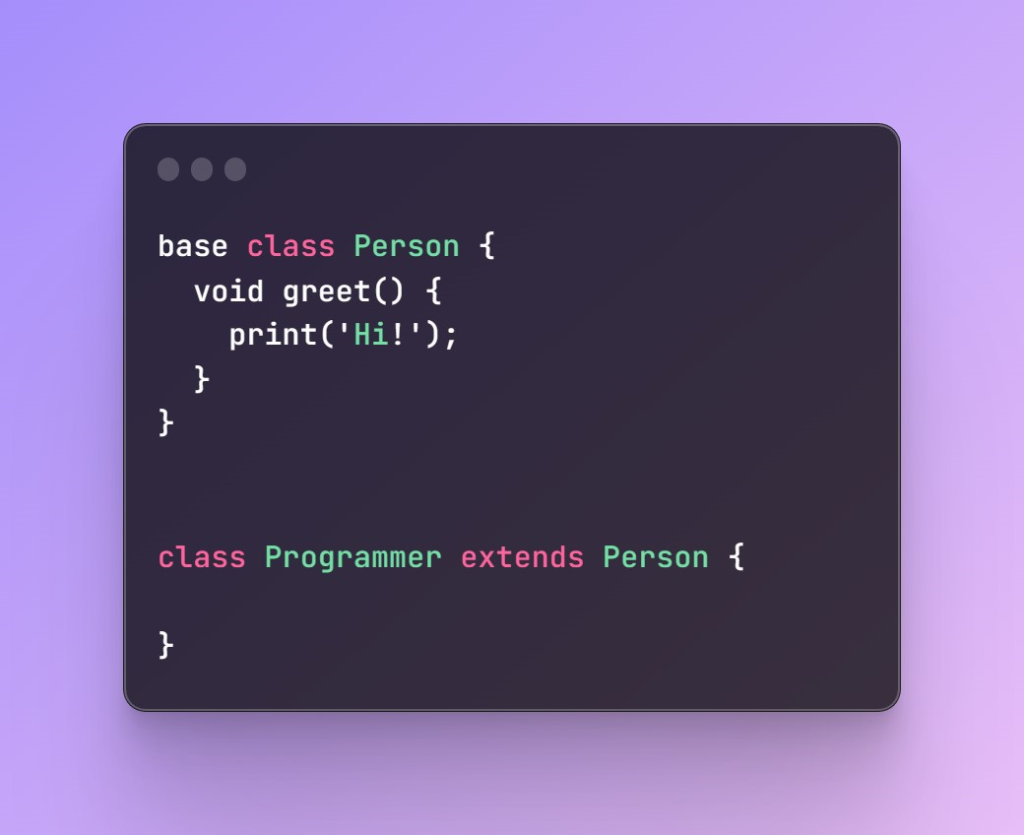
It will give the error like the following:
The type ‘Programmer’ must be ‘base’, ‘final’ or ‘sealed’ because the supertype ‘Person’ is ‘base’.
The base modifier works like a reverse version of the interface keyword. You can only extend your base class in another library. But keep in mind that if you want to extend the base class, your subclass should define as a base or final class.
Sealed Modifier
It allows us to create an enumerable set of subtypes. Using the new exhaustive check feature We can use subtype classes in switch and switch expressions anywhere with more friendly syntax.
The sealed modifier prevents a class from being extended or implemented outside its library.
Sealed classes can’t be instantiated, but they can contain factory constructors. So, it is implicitly abstract.
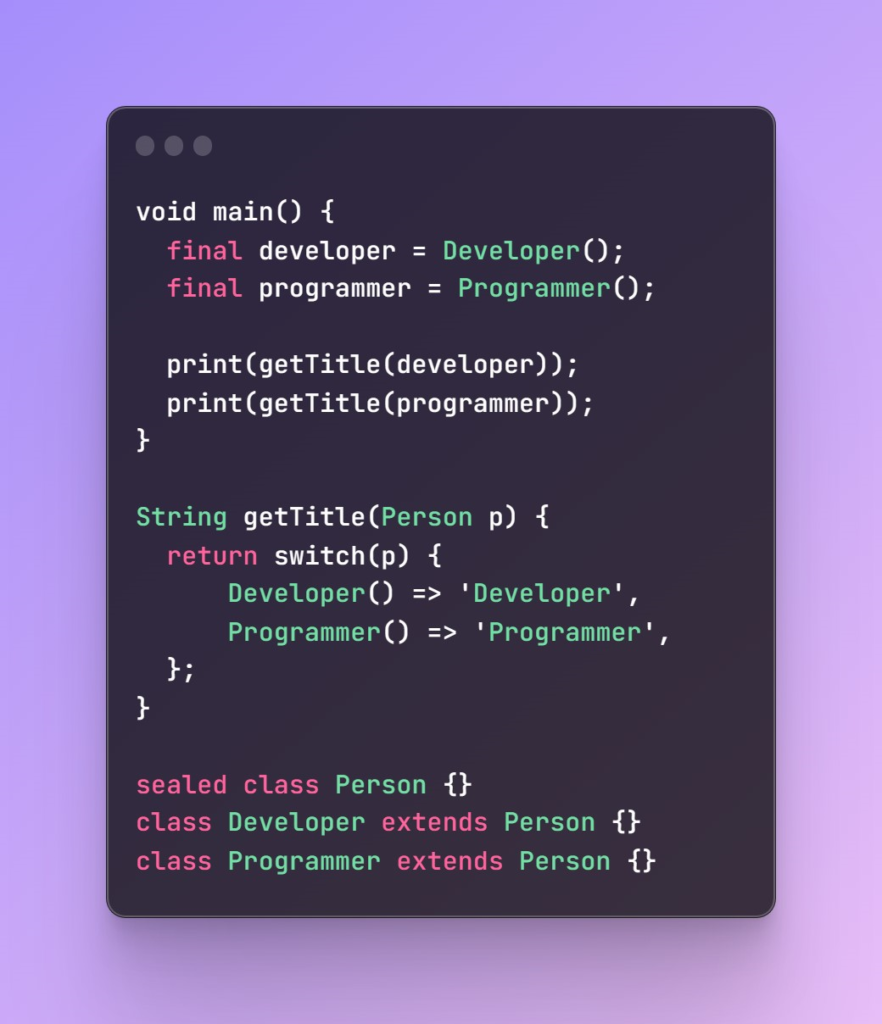
You can use sealed classes to handle different states in state management solutions (like Bloc, Riverpod, etc) in a more readable way:
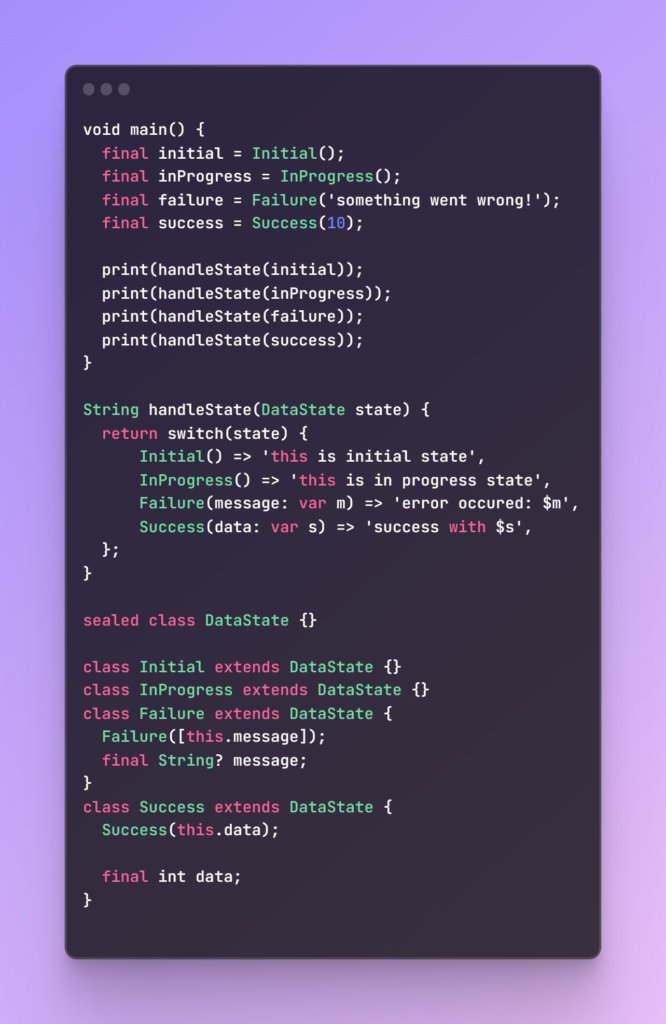
Combining modifiers
You can follow the following order to add class modifiers:
- in the first place, you can add the abstract keyword, but it is optional, it will allow the class to add some abstract members and will block the class to instantiate.
- Then, you can add one of the following keywords interface, final, base, and sealed to add more rules for subclasses in other libraries. (it is also optional)
- By the order, you can add a mixin keyword to add the capability for other classes to mix in. (optional)
- and it is required to add the class keyword itself.
For some types, you can’t combine. Keep in mind that:
- You can’t combine sealed and abstract classes, because the sealed class is implicitly abstract.
- You can’t use mixin with final,interface, and sealed, because they can’t allow other libraries to use them as mixin.
I hope it will be informative article for you.